I’m a good comic reader since I was young. I have a lot of comics, mainly Marvel, and I would like a way to easely catalog them a browse when needed. For this reason I would like to create an app where to add and catalog them. Let’s start then from the smaller concept possible: a table on a datatbase (SQL Server) defining a first embrional concept of Comic like the below:
- ID: identifier unique
- Series: name of the Comic serie
- Title: title of the specic comic
- Number: progressive number of the comic within the Series
I’m creating this on an empty SQL Database calog and in a Table named “Comic”
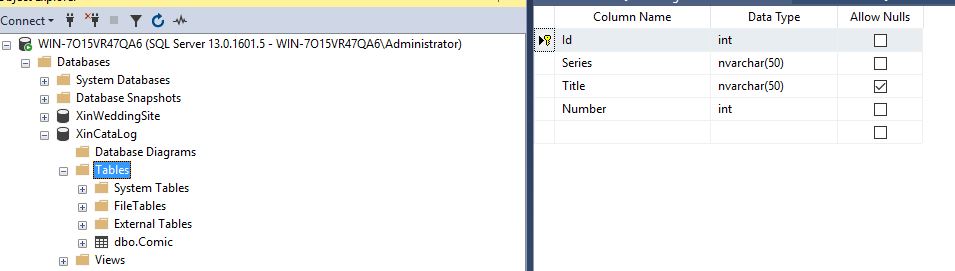
Let’s move now to VisualStudio 2019 IDE to create the Web API Project
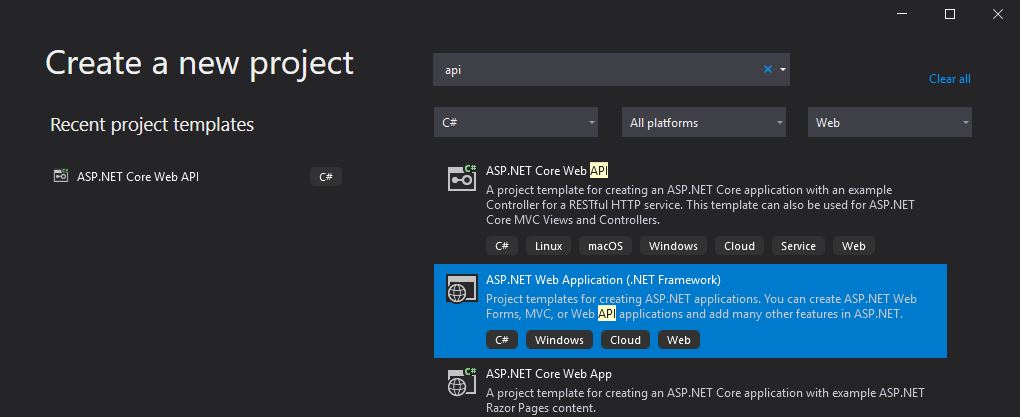
I will name the project XinCataLogAPI
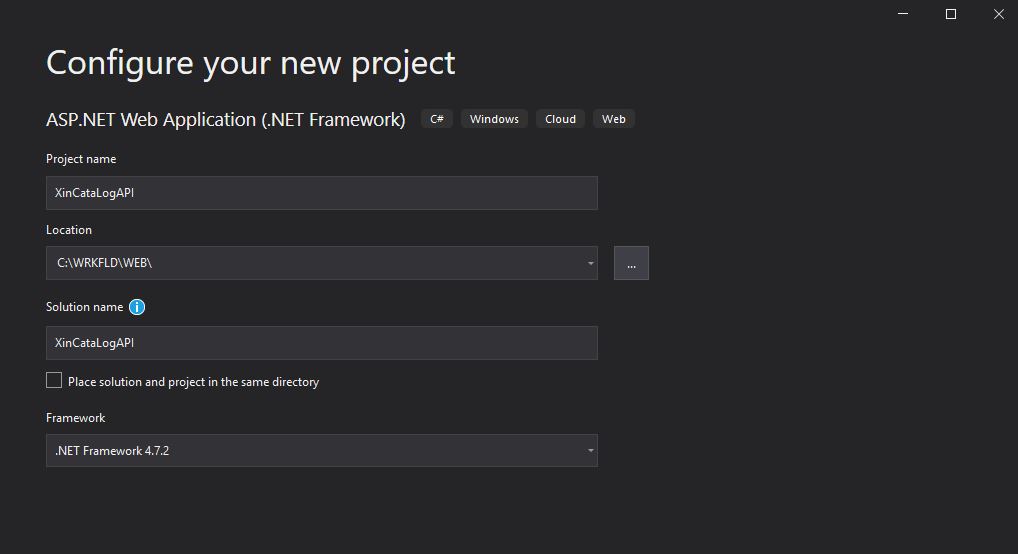
As target Framework I’m chosing 4.7.2 the latest one, for the template we need to choose Web API with no authentication (I saw in a lot of example this is not used, maybe I’m wrong).
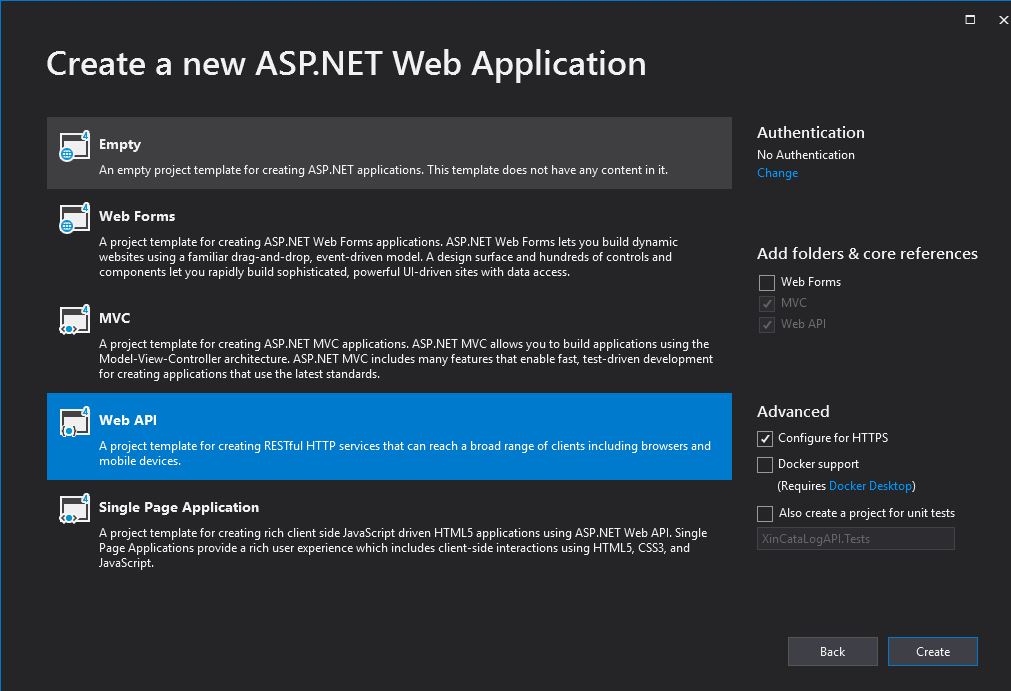
Ok, now the empty projet is created, I’ll build the project and run it in debug and voilĂ , the default WebApp is running.
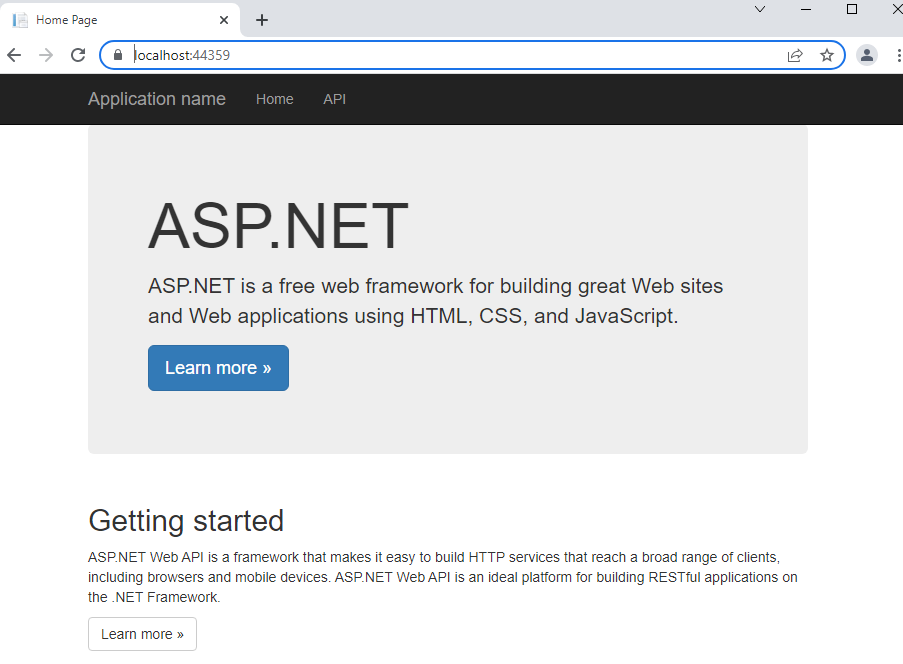
let’s add the project to the GitHub repository to ensure have a Source Control repository where to push and pull the versions.
Since we need to play with the EntityFramework to retrieve and manage the CRUD operation I’ll add a new Item to the solution under the MODEL Folder chosing the “ADO.NET Entity Data Model” and I’ll name it DBModel
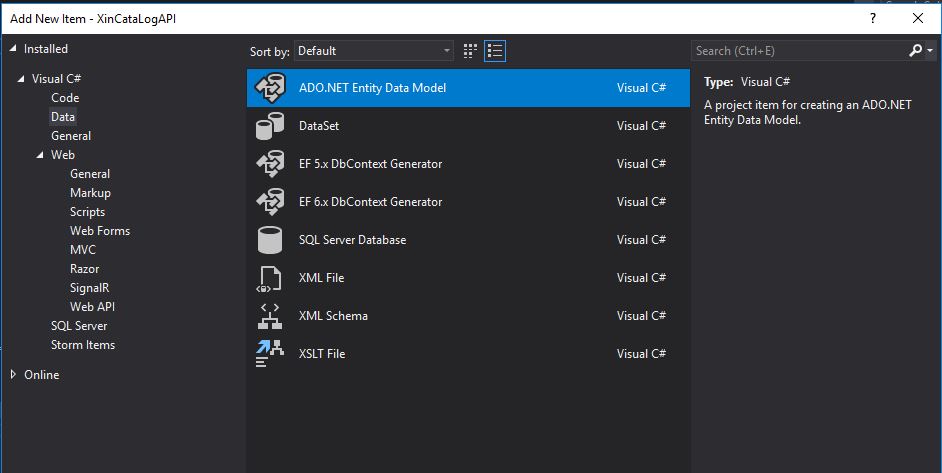
Now in the wizard which is opening let’s chose the Database First option:
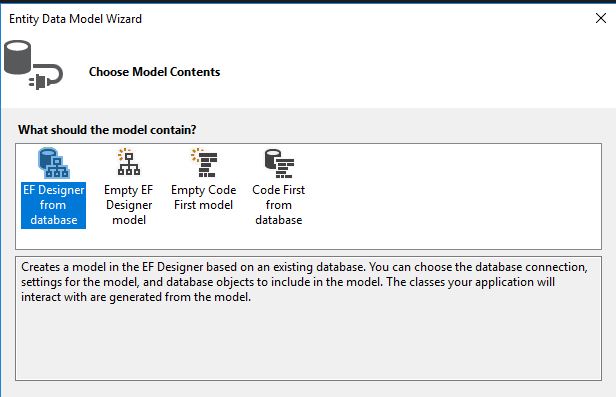
In the modal opening select the DB
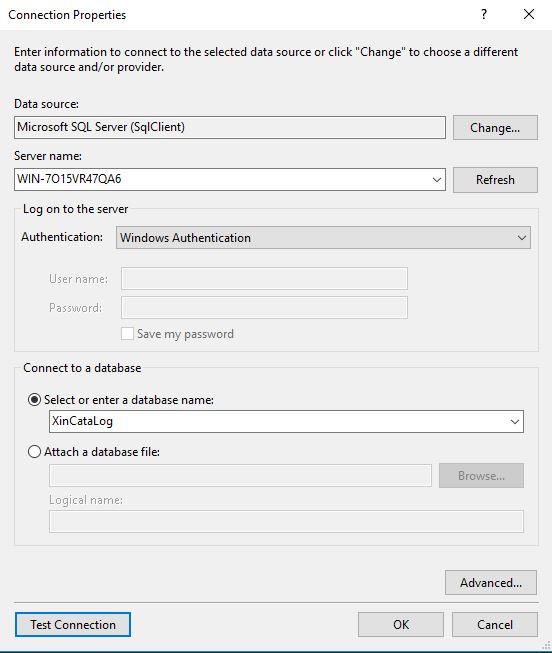
As option I chose the Entity Framework 6.x and then from the list of the object the Table XinComic:
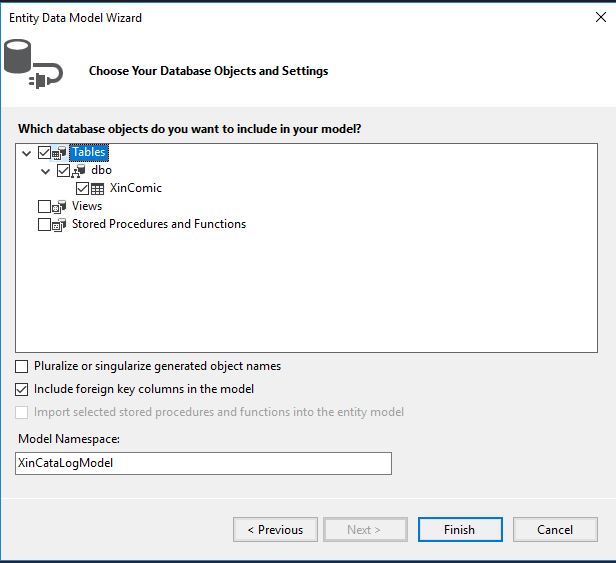
Once pressed Finish the Diagram will popup showing the object selected and will generate a set of items under the Model Folder.
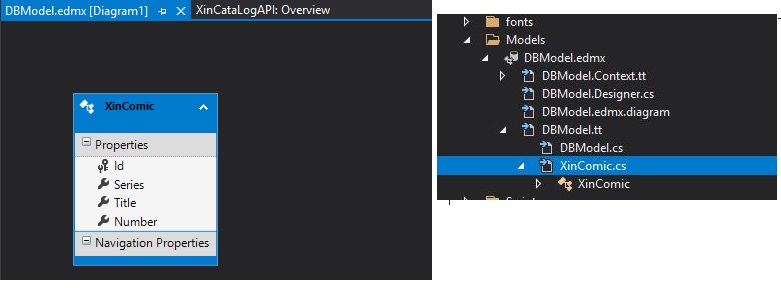
Before move ahead let’s spend a minute on two of the classes generated by the wizard:
- DBModel.Context.cs class is the one which is used to communicate with the Database
- XinComic.cs is the entity representing the Database table XinComic
Now that we have the classes which model the DB and create the DB we can move ahead. Tipically an API can provide the basic functionality to access to Database objects and more in details the four main HTTP methods (GET, PUT, POST, and DELETE) can be mapped to CRUD operations as follows (see also here [2]):
- GET retrieves the representation of the resource at a specified URI. GET should have no side effects on the server.
- PUT updates a resource at a specified URI. PUT can also be used to create a new resource at a specified URI, if the server allows clients to specify new URIs. For this tutorial, the API will not support creation through PUT.
- POST creates a new resource. The server assigns the URI for the new object and returns this URI as part of the response message.
- DELETE deletes a resource at a specified URI.
Since we have already a Model class created as above we can now move to create a Controller to implement the functionality above. To this let’s click on the Controller Folder and add a new controller like below
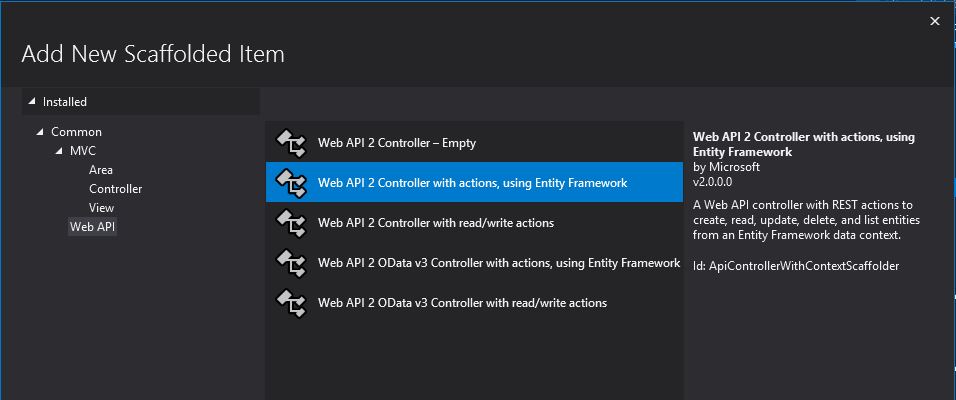
In the modal opening I just need then to specify the Model and the Repository created before
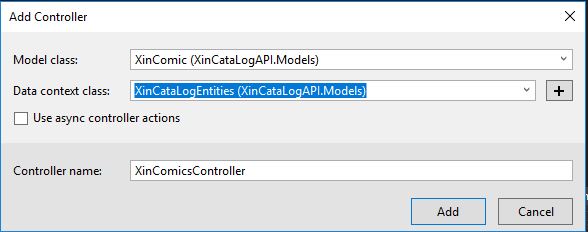
Then, after some actions from the wizard you should get the Controller correctly created: now in the controller folder I have a all the CRUD operation for my Comic:
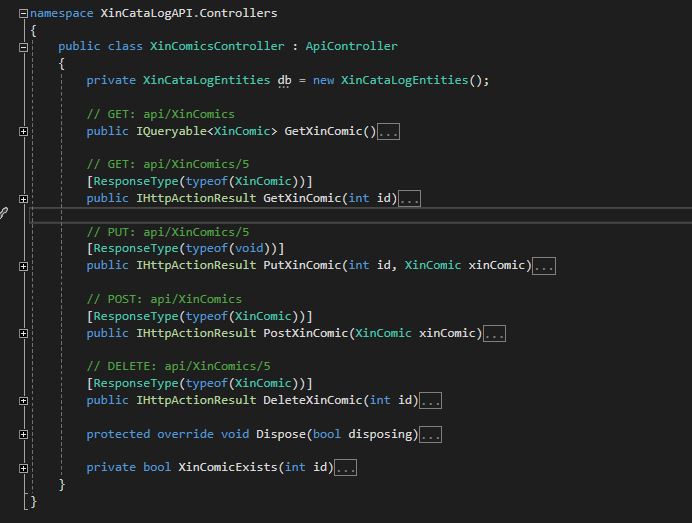
Ok, looks good, now if we rebuild the project, pressing the API top link we are direct to a page containing the list of the methods available:
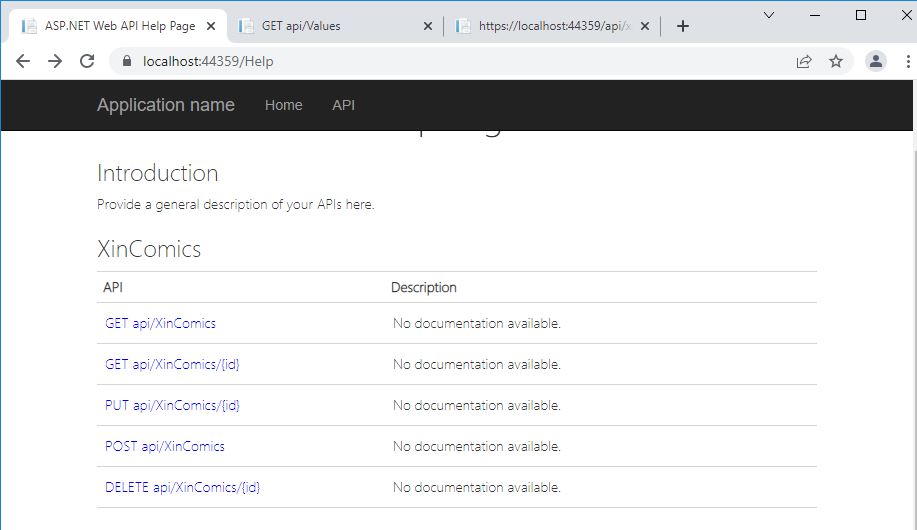
If we now put the url of the Get Method we can test the functionality:
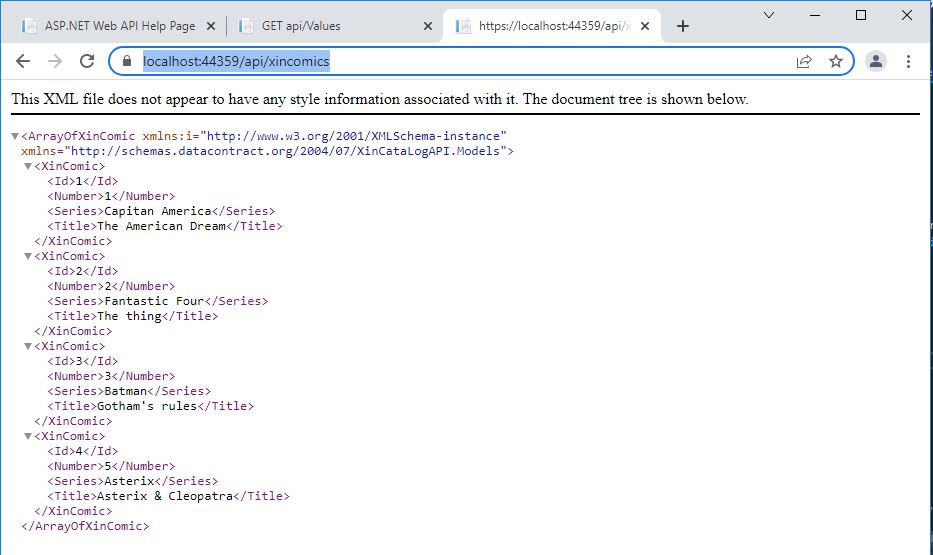
That is great but if we want to test the methods which requires a body it is not so straightful. In this case you may need to use an tool like Postman or Reqbin which can so the request to simulate the payloads like you can see below:
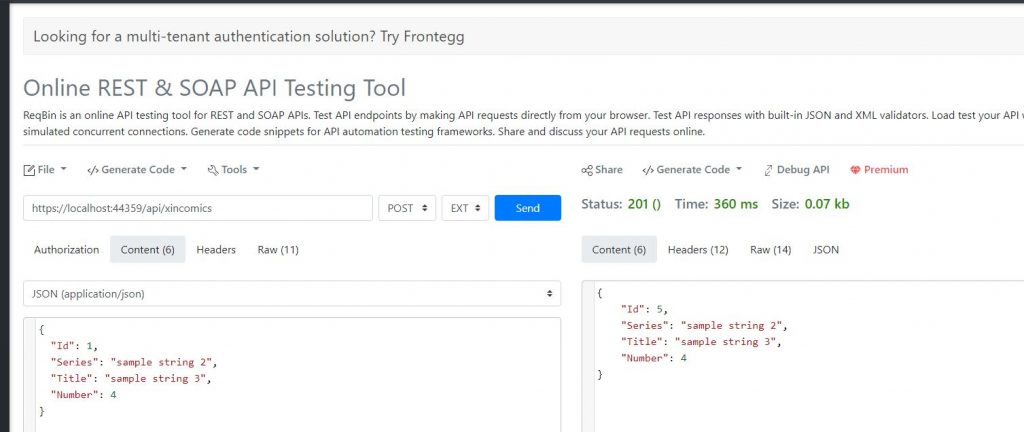
This can prove you the service is correctly replying. Another possibility, which a I prefer, is to leverage on Swagger to have a very intuitive way to test the API. I f you are interested please have a look to this post [4]. If you would have a look to this code please check this out [5] in my GitHub repository.
[3] https://stackoverflow.com/questions/45139243/asp-net-core-scaffolding-does-not-work-in-vs-2017
[4] https://www.beren.it/en/2022/03/26/comic-catalog-my-first-swagger-web-api/
[5] https://github.com/stepperxin/XinCataLogAPI